Easily Add Data to Excel UserForm ComboBox
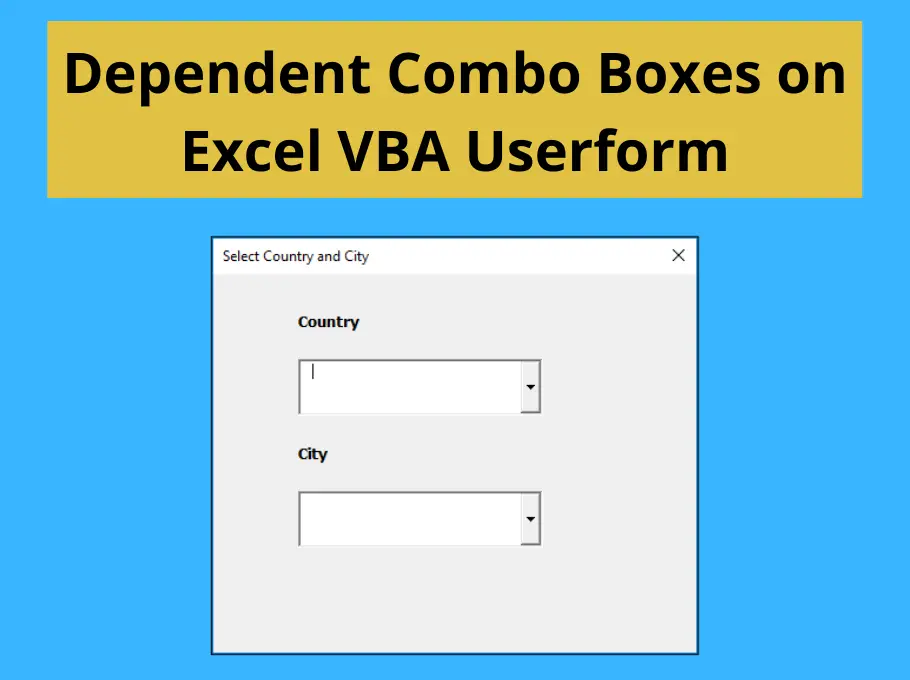
In today's world where data entry and management are critical, using tools like Excel UserForm for collecting and organizing data efficiently can be a game-changer. One of the most dynamic features you can implement in an Excel UserForm is a ComboBox which allows users to select items from a dropdown list. This post will guide you through the process of adding data to an Excel UserForm ComboBox with step-by-step instructions, tips, and best practices to ensure your form works seamlessly.
Understanding Excel UserForm
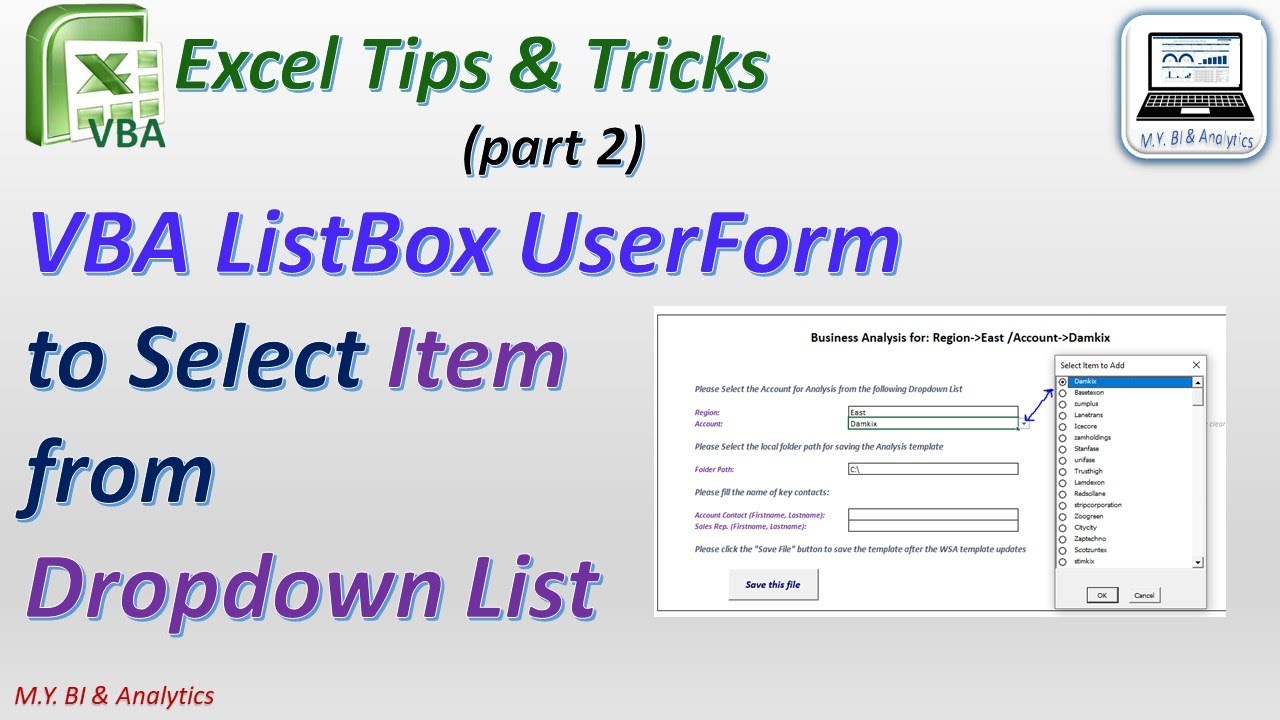
Before diving into adding data to a ComboBox, it's essential to understand the basics of Excel UserForms:
- What is an Excel UserForm? It's a custom dialog box where users can interact with VBA scripts in Excel. UserForms can contain various controls like buttons, labels, and input boxes.
- Why Use a UserForm? They provide a user-friendly interface for data entry, reducing errors, and streamlining the data collection process.
Setting Up Your Excel UserForm
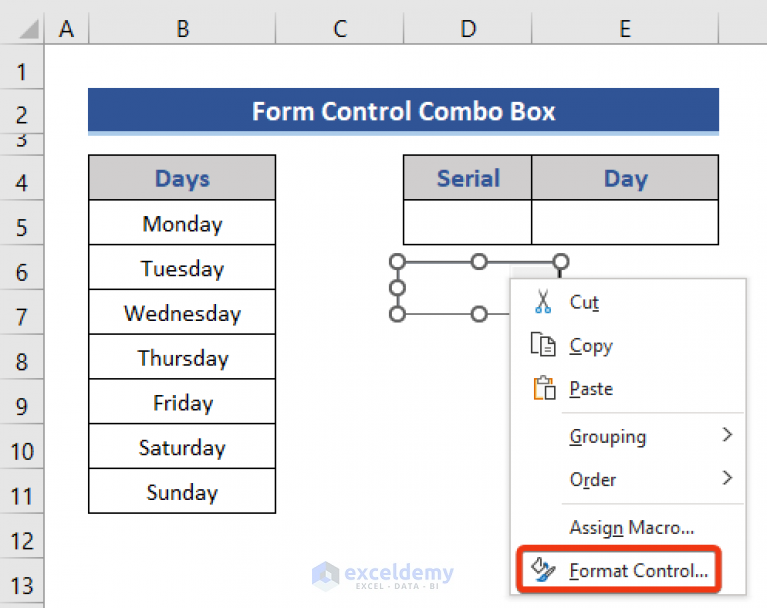
To begin:
- Open Excel and press Alt + F11 to open the VBA editor.
- In the VBA Editor, right-click on VBAProject (YourWorkbookName), select Insert > UserForm.
- From the toolbox, drag and drop a ComboBox onto your UserForm.
๐ Note: If the toolbox isn't visible, click View > ToolBox or press Ctrl + T.
Adding Data to the ComboBox
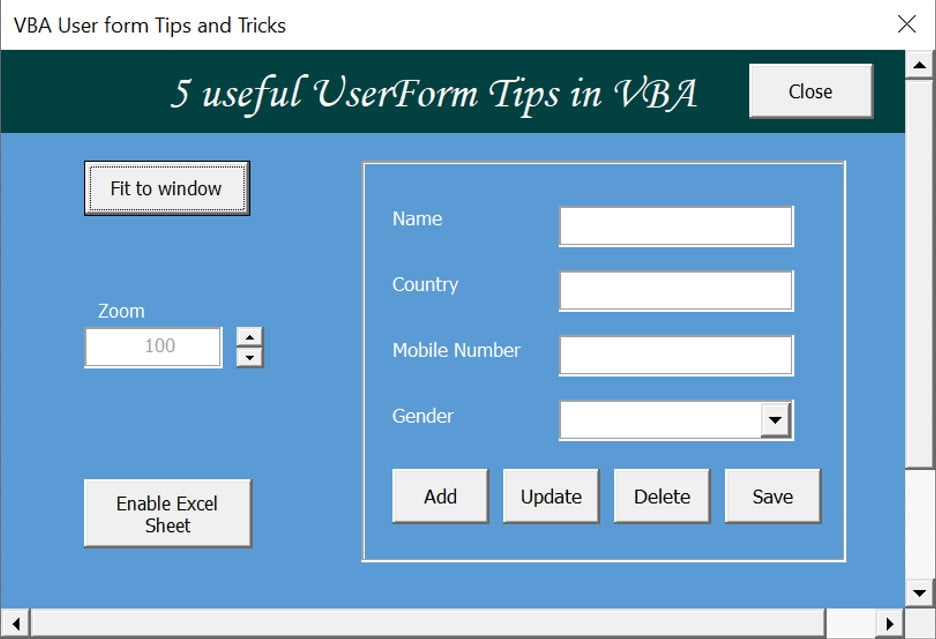
There are several methods to add data to your ComboBox:
Method 1: Manually Adding Items
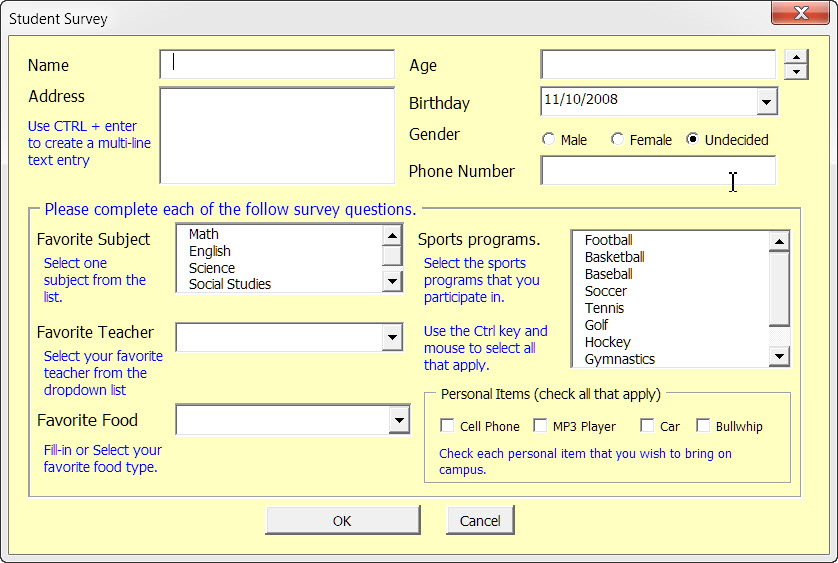
This approach is straightforward if you have a small, static list:
Private Sub UserForm_Initialize()
' Add items to the ComboBox one by one
Me.ComboBox1.AddItem "Item 1"
Me.ComboBox1.AddItem "Item 2"
Me.ComboBox1.AddItem "Item 3"
End Sub
This code snippet initializes the UserForm with preset items when it loads.
Method 2: Using a Range in Excel
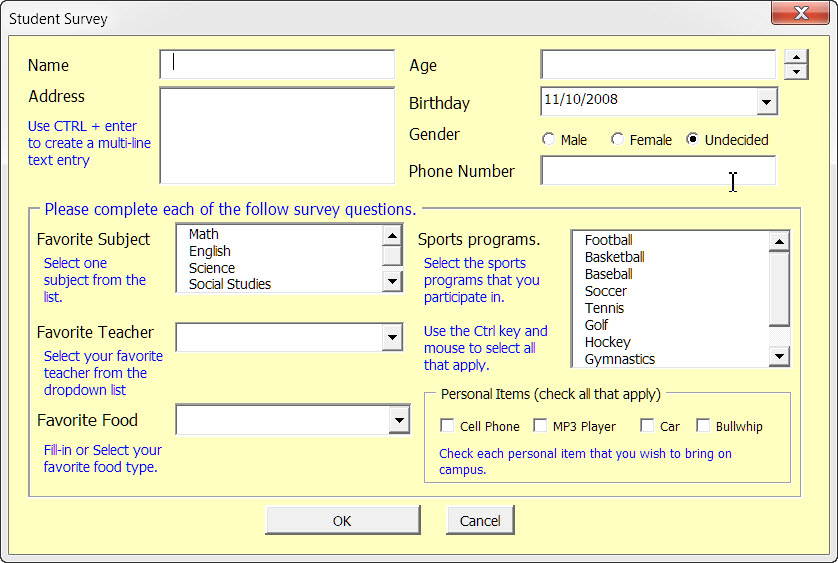
If your list of items is already in your worksheet:
Private Sub UserForm_Initialize()
Dim ws As Worksheet
Set ws = ThisWorkbook.Sheets("Sheet1")
With Me.ComboBox1
.List = ws.Range("A1:A5").Value
End With
End Sub
Here, the ComboBox is populated with values from range A1 to A5 in Sheet1.
Method 3: Adding from an Array
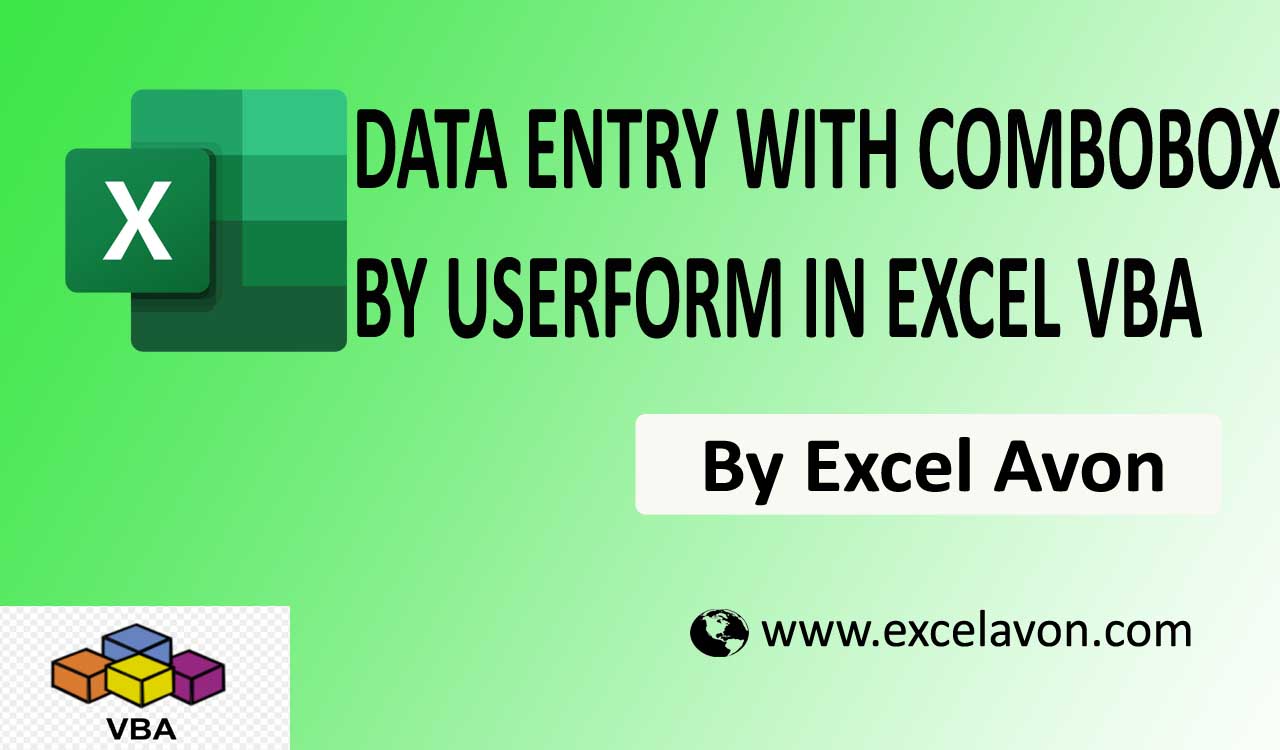
For dynamic data sets:
Private Sub UserForm_Initialize()
Dim Items(3) As String
Items(0) = "First Item"
Items(1) = "Second Item"
Items(2) = "Third Item"
Items(3) = "Fourth Item"
Me.ComboBox1.List = Items
End Sub
๐ Note: Ensure the array size matches the number of items you want to add.
Adding Event Handlers
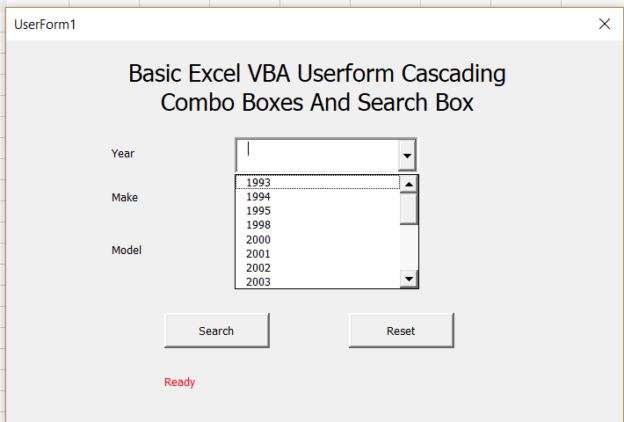
To make your ComboBox more interactive, you can add event handlers:
- Change Event: Triggers when the user selects an item from the list.
- GotFocus: Runs when the ComboBox receives focus.
Private Sub ComboBox1_Change()
MsgBox "You selected: " & Me.ComboBox1.Value
End Sub
Private Sub ComboBox1_GotFocus()
Me.ComboBox1.Clear
' Or any other initialization code
End Sub
Managing Multiple ComboBoxes
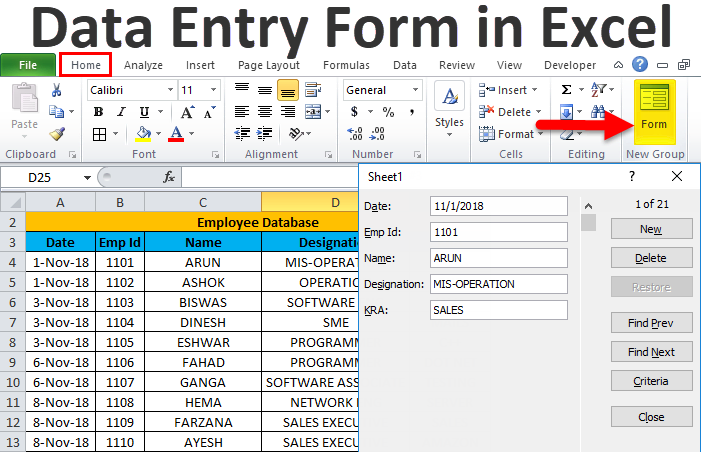
If your form has several ComboBoxes, you'll need to manage them efficiently:
- Give each ComboBox a unique name for easier VBA code referencing.
- Implement a control array if dealing with many similar ComboBoxes.
Implementing a Control Array
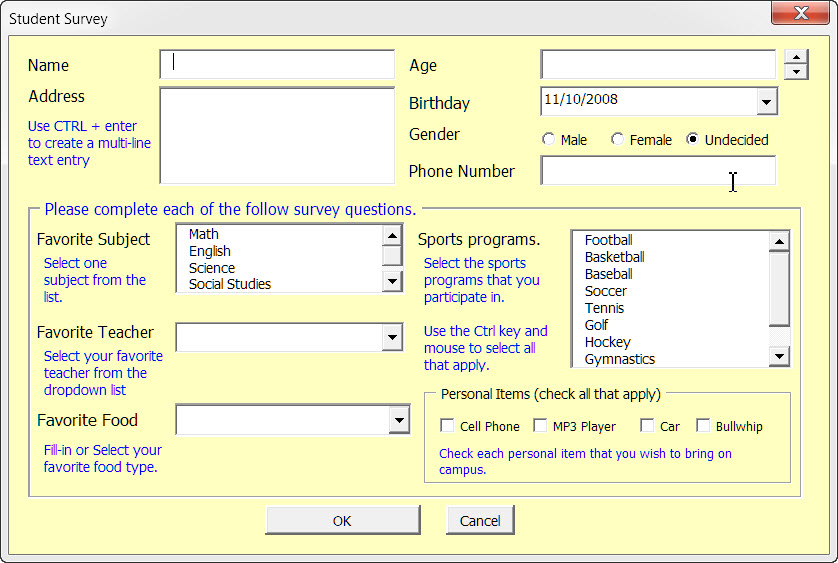
In VBA, you can create a control array as follows:
Private Sub UserForm_Initialize()
Dim i As Integer
For i = 1 To 5
Dim cbo As Control
Set cbo = Me.Controls.Add("Forms.ComboBox.1", "ComboBox" & i)
With cbo
.Left = 100
.Top = 20 + (i - 1) * 20
.List = Array("Item1", "Item2", "Item3")
End With
Next i
End Sub
Final Touches for Seamless User Experience
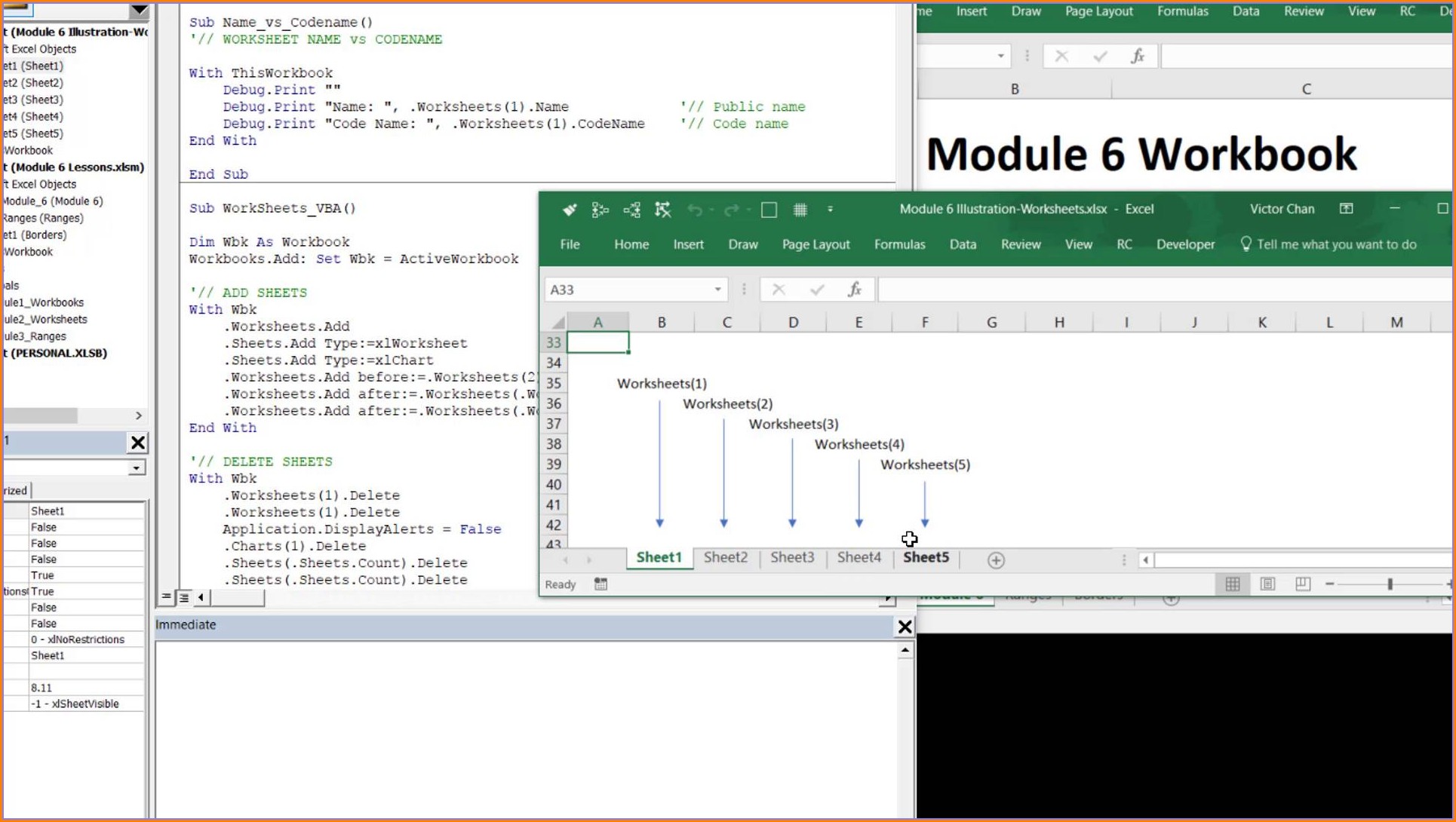
To ensure your UserForm is user-friendly:
- Resize ComboBoxes: Make sure your ComboBoxes are appropriately sized based on content.
- Sort Items: Add sorting functionality if necessary:
Private Sub ComboBox1_DropButtonClick()
With Me.ComboBox1
.List = SortArray(.List)
End With
End Sub
Function SortArray(arr As Variant) As Variant
Dim newArr() As String, i As Integer, temp As String
ReDim newArr(LBound(arr) To UBound(arr))
newArr = arr
For i = LBound(newArr) To UBound(newArr) - 1
For j = i + 1 To UBound(newArr)
If newArr(i) > newArr(j) Then
temp = newArr(i)
newArr(i) = newArr(j)
newArr(j) = temp
End If
Next j
Next i
SortArray = newArr
End Function
Recapping the Process
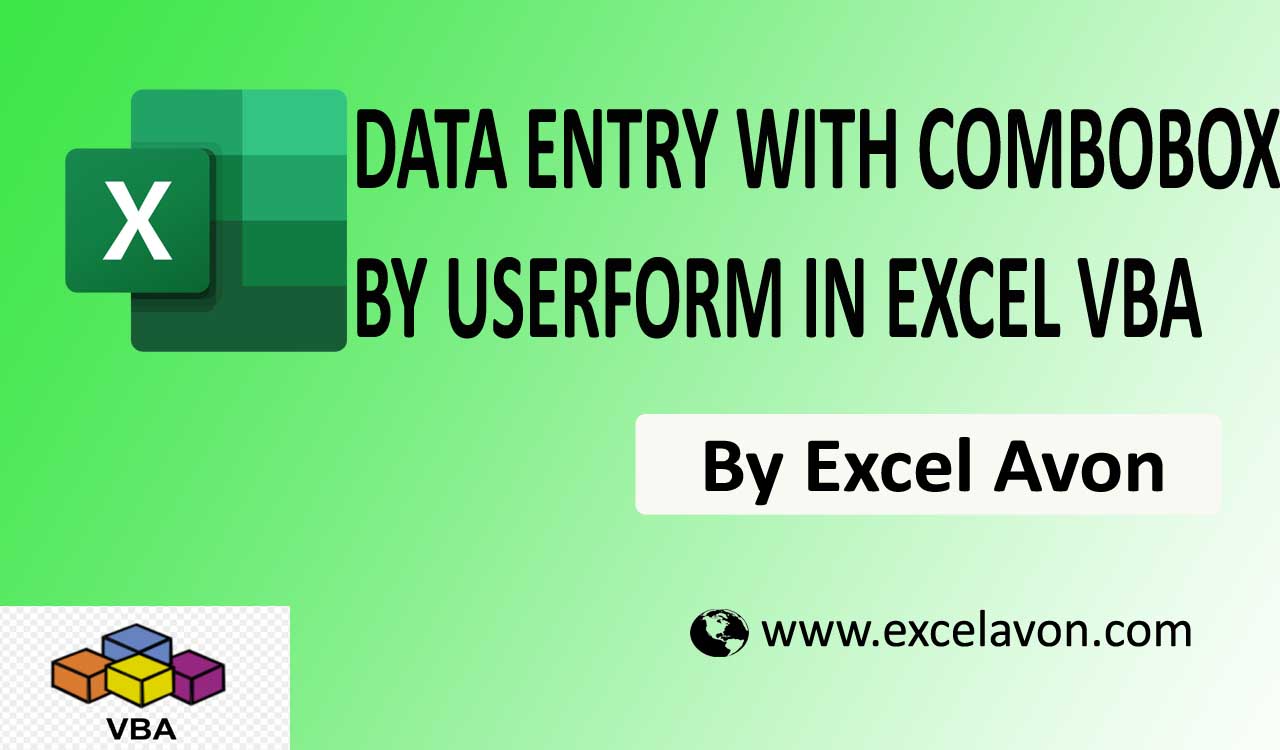
We've explored the process of adding data to an Excel UserForm ComboBox. From understanding the basics of UserForms to implementing dynamic data loading and managing multiple ComboBoxes, the key is to match your data management needs with the appropriate method for populating your ComboBox. By following these steps, you'll ensure your forms are not only functional but also user-friendly, enhancing data entry efficiency and accuracy in your Excel workflows.
How do I clear the items in a ComboBox?
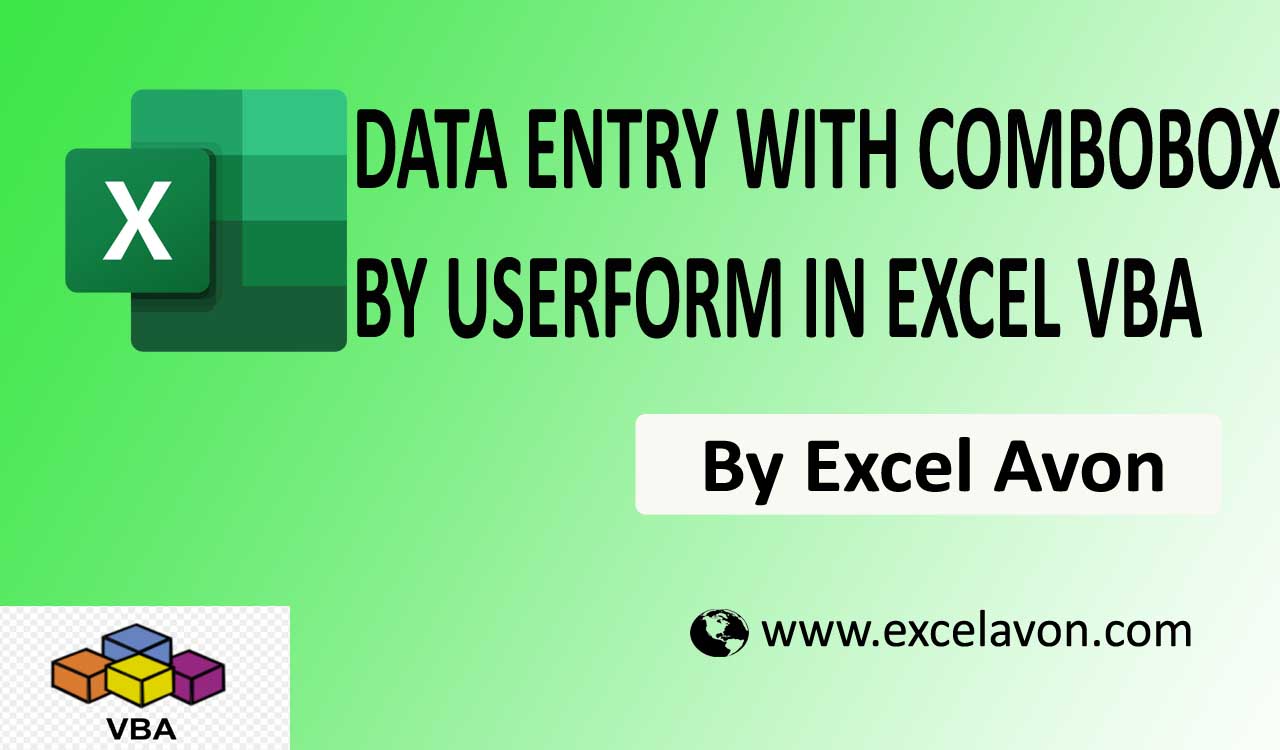
+
To clear the items in a ComboBox, you can use ComboBox1.Clear within your VBA code.
Can I add data to a ComboBox from another worksheet?
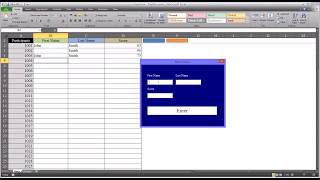
+
Yes, you can specify the worksheet in your VBA code like this: Set ws = ThisWorkbook.Sheets(โSheet2โ) before populating the ComboBox.
What if I need to load data dynamically?
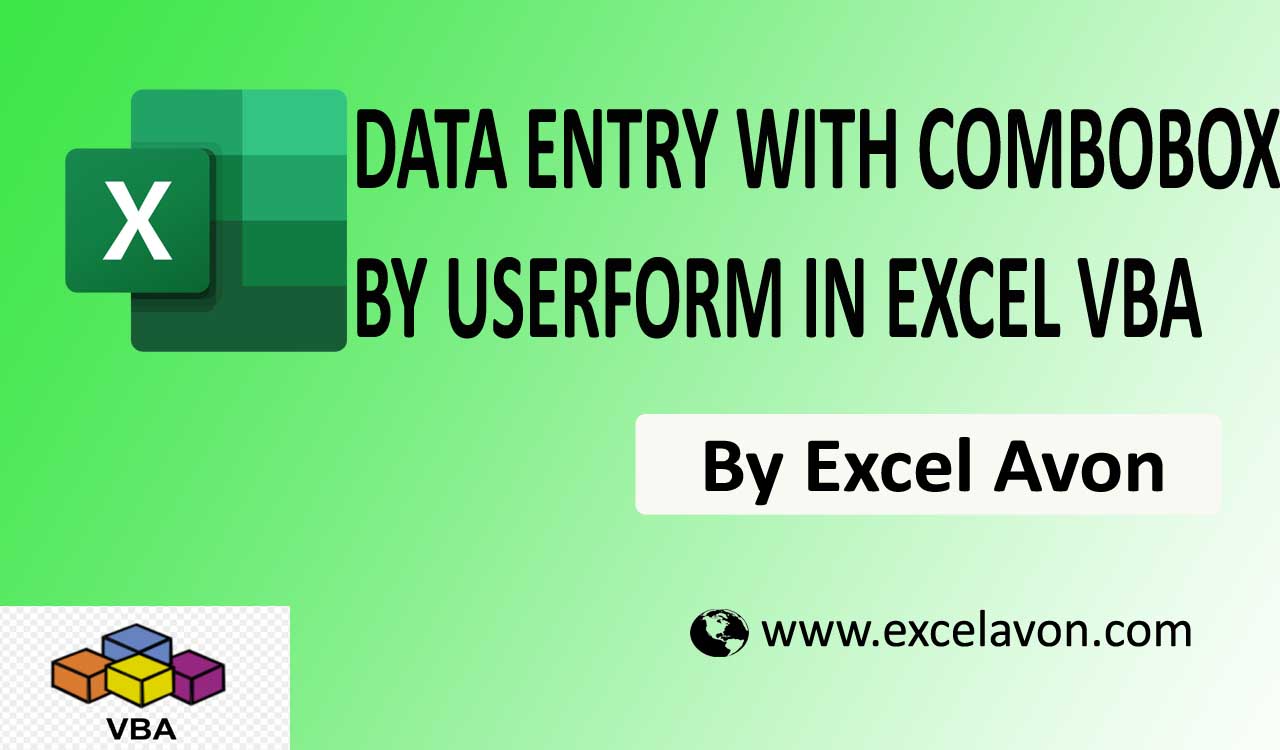
+
For dynamic data, you could either use an event like Worksheet_Change to trigger updates or design the UserForm to load data each time itโs opened.